How to Use Environment Variables in Node.js?
Working with Environment Variables is IMPORTANT in Software Development. Every stage, such as Development, Testing or Production, uses its environment variables. It also secures the Privacy for Production on clouds such as Heroku, AWS, Azure,… How do we do that in NodeJS?
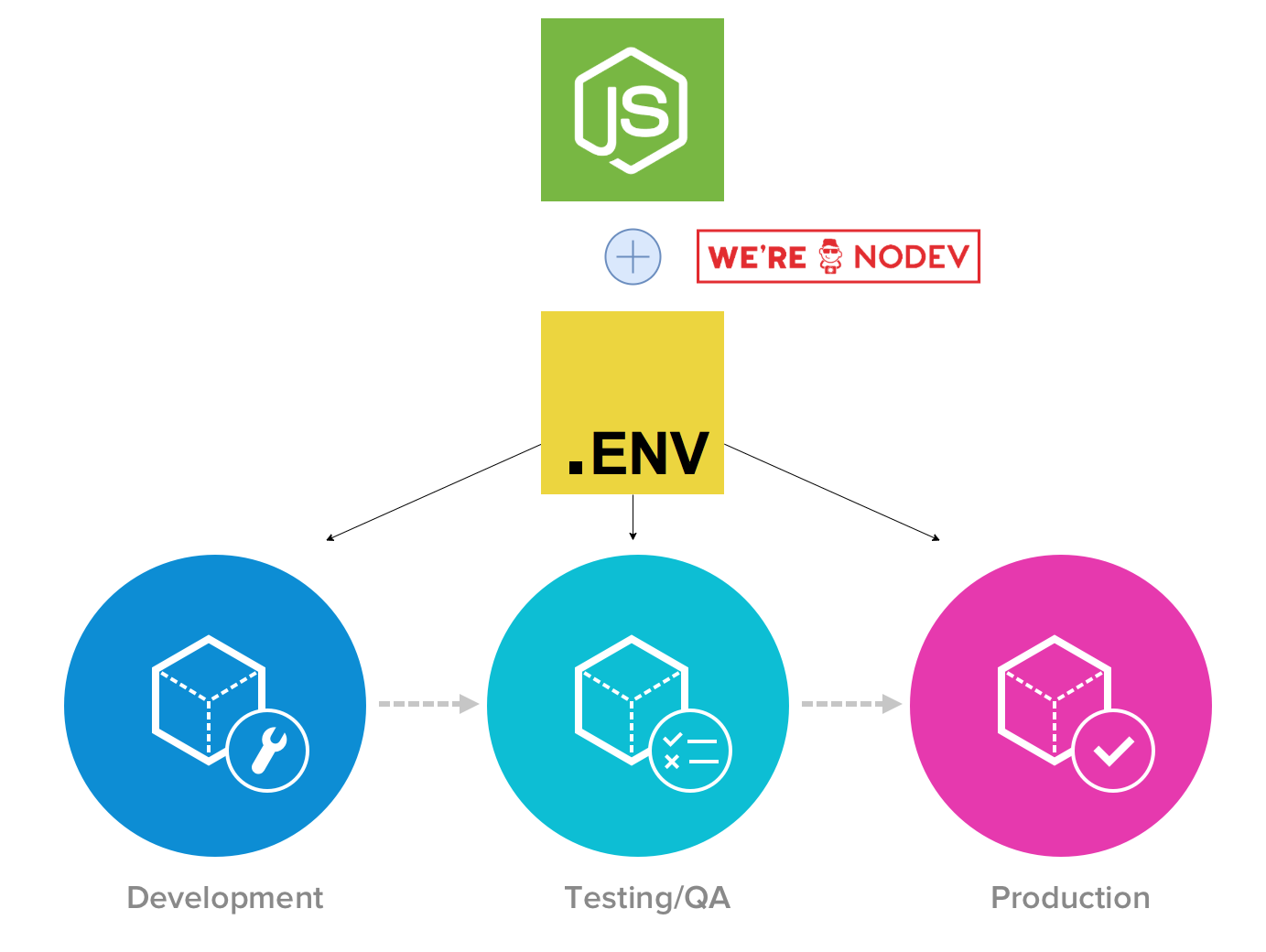
Introduction
As I mentioned above, suppose that we need to connect to a database, we maybe have a username
and a password
for connection. However, each environment our team use is different. Everyone in our team uses localhost
with own username
and password
. Password and Private Key are IMPORTANT and PRIVACY on production, which only someone has and use it on cloud or server. Therefore environment variables is neccessary when our team developes an application.
In this tutorial, I’ll introduce you how we can use environment variables in Node.js Application.
What is dotenv
?
dotenv - Loads environment variables from .env for nodejs projects.
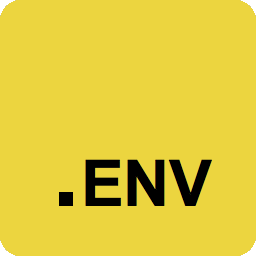
Dotenv is a zero-dependency module that loads environment variables from a .env file into process.env. Storing configuration in the environment separate from code is based on The Twelve-Factor App methodology.
In Node.js Application, we’ll use package dotenv
to connect environment variables.s
How to Use dotenv
in Node.js?
Create simple Node.js project
- Create directory
node-env
mkdir node-env
- Use
npm init
to create simple Node.js with filepackage.json
npm init
package.json
will be such as:
{
"name": "@sam/node-env",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
- Create file
index.js
:
touch index.js
- Edit file
index.js
with:
console.log('Hello Node.js');
- In
package.json
, add start script which run fileindex.js
:
{
"name": "@sam/node-env",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index",
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
- Start file
index.js
by:
npm start
- You will see on terminal
> node index
Hello Node.js
Install dotenv
dependency
yarn add dotenv
# or use with npm
npm install dotenv --save
Config, Load and Read Environment Variables
- In file
index.js
, at the top, require and configdotenv
:
require('dotenv').config();
- Create file
.env
and add some environment,dotenv
will load them toprocess.env.*
:
touch .env
In file .env
add some variables:
USERNAME=huynhsamha
PASSWORD=kjry9bq34vj0394u0349ut02v33v5y
- Edit file
index.js
:
require('dotenv').config();
console.log(process.env.USERNAME);
console.log(process.env.PASSWORD)
- You will see on terminal:
> node index
huynhsamha
kjry9bq34vj0394u0349ut02v33v5y
Ignore file .env
If you use git
for your project, file .env
should not included in repository, in file .gitignore
, add .env
to ignore it.
.env
You should use the default file .gitignore
for Node.js Application at https://github.com/github/gitignore/blob/master/Node.gitignore
Conclusion
I have shown you how to use environment variables in Node.js application with dotenv
.
Thanks for reading my article! If you have any feedback or criticism, feel free to leave any comment!